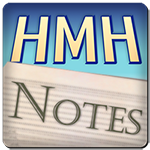
Handmade Hero Notes
Foreword -
Appendix. Glossary and References
Day 1. Setting Up the Windows Build
-
Set up Visual Studio.
- Configure Command Prompt.
-
Set up a Text Editor (4coder/vscode).
-
Create the First Code File (
win32_handmade.cpp
).
build.bat
philosophy.
-
Intro to debugging in
Visual Studio and
RemedyBG.
Day 2. Opening a Win32 Window
- Create and Register the WindowClass.
-
Create Main Windows Callback, Start Processing Basic Messages.
- Create a Window.
- Start the Message Loop with
GetMessageA
.
Day 3. Allocating a Back Buffer
- Create a Bitmap using
CreateDIBSection
.
- Output the Bitmap to the Window using
StretchDIBits
.
Day 4. Animating the Back Buffer
- Custom Bitmap Memory Allocation.
- Render a Gradient to the Bitmap.
- Animate the Bitmap on Each Frame.
- RGB Color Packing in Windows.
Day 5. Windows Graphics Review
- Pack the Bitmap-related Globals Into a Struct
- The Stack
- Full Program Step-through
Day 6. Gamepad and Keyboard Input
- XInput
- Direct Library Loading
- Process Keyboard Messages
Day 7. Initializing DirectSound
- Load Direct Sound
- Initialize the Buffers
- Deep Dive: COM, Vtables, Disassembly
Day 8. Writing a Square Wave to DirectSound
- Write to a Circular Buffer
- Square Wave
- Play Sound through DirectSound
Day 9. Variable-Pitch Sine Wave Output
- Generate a Sine Wave
- Debug Sample Writing
- Link Sound Pitch to User Input
Day 10. QueryPerformanceCounter and RDTSC
-
Time the frame using
QueryPerformanceCounter
and
RDTSC
- String formatting tools in the C Standard Library
- Intro to Dimensional Analysis
Day 11. The Basics of Platform API Design
- About Code Architecture
- Platform Usage Philosophies
- Start Implementing Game as Service System Style
Day 12. Platform-Independent Sound Output
- Intro to our API Design Approach
- Start Generating Sounds on the Game Layer
- Make Use of Sound Generation in the Platform Layer
Day 13. Platform-Independent User Input
- Think of a Possible Input System Usage
- Make a First Pass on the Input System
Day 14. Platform-Independent Game Memory
- Intro to Memory Management
- Design and Implementation of Our Approach
- Assertions
Day 15. Platform-Independent Debug Game I/O
- About File I/O in Games
- Platform Implementation of the Debug I/O
- Safe Truncation
Day 16. Visual Studio Compiler Switches
- Enable Compiler Warnings
- Set Other Compiler Switches
- Try a 32-bit Build
Day 17. Unified Keyboard and Gamepad Input
- Move Keyboard Input to WinMain
- Finish Gamepad Stick Code
- Improve Keyboard Input Processing
Day 18. Enforcing a Video Frame Rate
- Define frame rate problem
- Implement first pass solution
- Some Cleanup and Refactoring
Day 19. Improving Audio Synchronization
- Visualize the DirectSound Cursors
- Deep-dive Debugging: Audio Skipping
- Increase Audio Latency
Day 20. Debugging the Audio Sync
- Deep Dive into Audio Latency Issue
- Implement Two Audio Paths
- Introduce GameGetSoundSamples
- More Debug Work
Day 21. Loading Game Code Dynamically
- Separate Game From Platform
- Build Game DLL
- Reload Game DLL at Runtime
Day 22. Instantaneous Live Code Editing
- Variable PDB Name
- Re-enable Debugger Support
- Remove Delay Between Reloads
Day 23. Looped Live Code Editing
- Add a Test Player
- Implement Live Loop
- Showcase Live Loop Editing
Day 24. Win32 Platform Layer Cleanup
- Various Code Improvements
- String Building Routines
Day 25. Finishing the Win32 Prototyping Layer
- Capture Mouse Input
- Improve Replay Handling
- Clean Up Platform Debug Code
Day 26. Introduction to Game Architecture
- About Software Architecture
- Game Architecture for Handmade Hero
Day 27. Exploration-based Architecture
- Explore and Lock architecture approach
- Game Design for Handmade Hero
- Test Code Cleanup
- DrawRectangle Function
- Floating-point Color
- Basic Tile Map
- Getting a Player on the Screen
- Aside: A bit of Color Theory
Day 29. Basic Tile Map Collision Checking
- Player Collision with the Tilemap
- Multiple Tile Maps